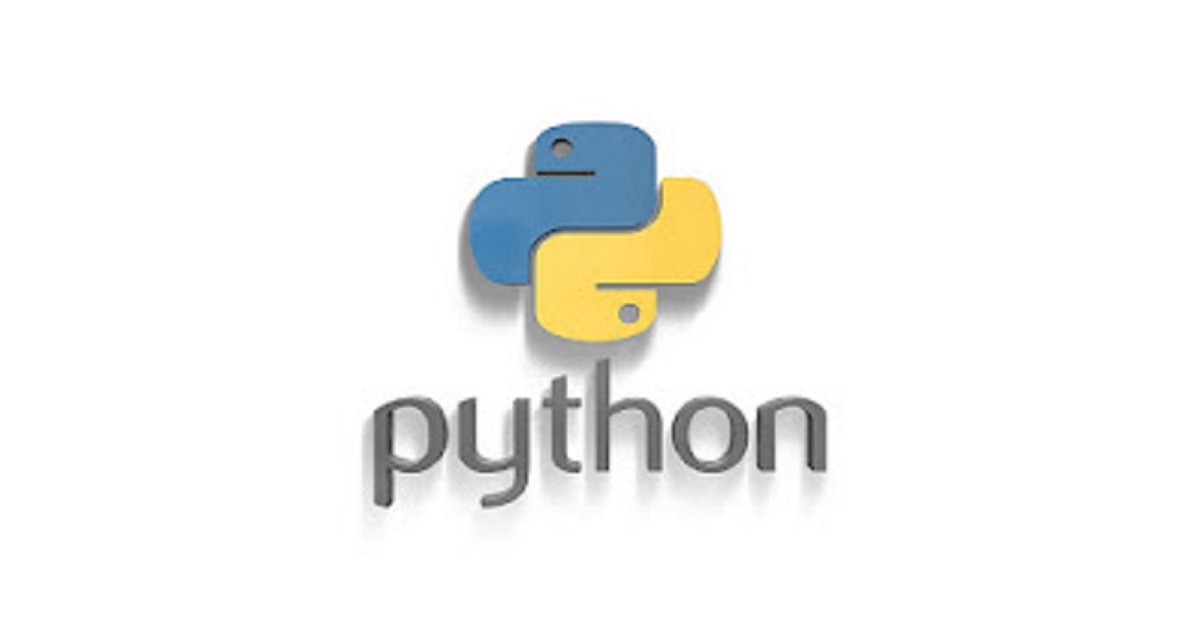
Python has become one of the most popular programming languages in the world due to its simplicity and versatility. Whether you are a beginner or an experienced developer, Python offers an intuitive syntax and powerful libraries that make it suitable for a wide range of applications, from web development to data science and artificial intelligence. This guide, “Digiskool: a Practical Introduction to Python Programming,” aims to provide you with a hands-on approach to learning Python, equipping you with the foundational skills needed to build your projects.
Chapter 1: Getting Started with Python
Installing Python
Before you can start programming in Python, you need to install it on your computer. Python is available for all major operating systems, including Windows, macOS, and Linux. Visit the official Python website and download the latest version. Follow the installation instructions provided for your operating system.
Setting Up a Development Environment
Once Python is installed, you will need a code editor to write your Python scripts. Some popular code editors include:
- Visual Studio Code (VS Code)
- PyCharm
- Sublime Text
- Jupyter Notebook
Choose an editor that you feel comfortable with and set it up according to your preferences.
Chapter 2: Basic Python Syntax
Hello, World!
Let’s start with a simple “Hello, World!” program. Open your code editor and create a new file called hello.py
. Enter the following code:
python
print("Hello, World!")
Save the file and run it using the command line:
bash
python hello.py
You should see the output:
Hello, World!
Variables and Data Types
Python supports various data types, including integers, floats, strings, and booleans. Here is how you can use them:
python
# Integer
x = 5
# Float
y = 3.14
# String
name = “Alice”
# Boolean
is_student = True
Basic Operations
You can perform basic arithmetic operations in Python:
python
# Addition
sum = x + y
# Subtraction
difference = x – y
# Multiplication
product = x * y
# Division
quotient = x / y
Chapter 3: Control Structures
Conditional Statements
Python uses if
, elif
, and else
statements to control the flow of the program based on conditions:
python
if x > y:
print("x is greater than y")
elif x < y:
print("x is less than y")
else:
print("x is equal to y")
Loops
Python provides for
and while
loops to iterate over sequences and perform repetitive tasks:
python
# For loop
for i in range(5):
print(i)
# While loop
count = 0
while count < 5:
print(count)
count += 1
Chapter 4: Functions
Defining Functions
Functions are reusable blocks of code that perform a specific task. You can define a function using the def
keyword:
python
def greet(name):
print(f"Hello, {name}!")
greet(“Alice”)
Returning Values
Functions can also return values using the return
keyword:
python
def add(a, b):
return a + b
result = add(5, 3)
print(result)
Chapter 5: Data Structures
Lists
Lists are ordered collections of items. You can create and manipulate lists as follows:
python
fruits = ["apple", "banana", "cherry"]
fruits.append("orange")
print(fruits)
Dictionaries
Dictionaries store key-value pairs and provide a way to organize data:
python
student = {"name": "Alice", "age": 20, "major": "Computer Science"}
print(student["name"])
Tuples and Sets
Tuples are immutable sequences, and sets are unordered collections of unique items:
python
# Tuple
coordinates = (10, 20)
# Set
unique_numbers = {1, 2, 3, 3, 4}
Chapter 6: Modules and Packages
Importing Modules
Modules are files containing Python code that can be imported into other scripts. Python comes with many built-in modules:
python
import math
print(math.sqrt(16))
Creating Packages
You can organize your code into packages by creating directories with __init__.py
files. This helps manage larger projects.
Chapter 7: File Handling
Reading and Writing Files
Python provides built-in functions to read from and write to files:
python
# Writing to a file
with open("example.txt", "w") as file:
file.write("Hello, World!")
# Reading from a file
with open(“example.txt”, “r”) as file:
content = file.read()
print(content)
Conclusion
This guide has introduced you to the basics of Python programming, from setting up your development environment to writing and running simple scripts. With these foundational skills, you are ready to explore more advanced topics and build your projects. Remember, practice is key to becoming proficient in programming. read more Digiskool.